스프링에서 비동기 통신이 발생할 때 사용하는 애노테이션들이다. 참고로 웹에서 화면전환(새로고침) 없이 이루어지는 동작들은 대부분 비동기 통신으로 이뤄진다.
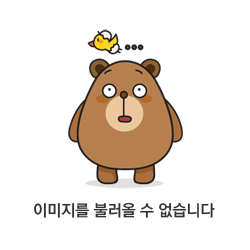
1. @RequestBody
- HTTP Request Body의 JSON, XML, Text 등의 데이터를 적합한 HttpMessageConverter를 통해 파싱하여 Java 객체로 변환한다.
- POST 요청에서 JSON을 Java 객체로 변환해주는 것은 Jackson2HttpMessageConverter이기 때문에, setter 메서드가 필요 없다.
- GET 요청의 경우에는 JSON이 아닌 쿼리 파라미터로 데이터를 받기 때문에, Jackson2HttpMessageConverter가 아닌 스프링의 WebDataBinder를 사용한다. WebDataBinder는 자바빈 방식이 기본 설정이기 때문에 setter 메서드가 필요하다.
- 대부분의 경우 기본 생성자가 필요하다.
@RestController
@RequestMapping("/api")
public class UserController {
@GetMapping("/user")
public String getUser(@RequestBody User user) {
UserService.getUserByObject(user);
return "ok";
}
}
2. @ResponseBody
- Java 객체를 HTTP Message Body에 직접 담아서 전달할 수 있다.
/**
@RestController = @Controller + @ResponseBody로 볼 수도 있다.
**/
@Controller
@ResponseBody
@RequestMapping("/api")
public class UserController {
@GetMapping("/user")
public String getUser(@RequestBody User user) {
UserService.getUserByObject(user);
return "ok";
}
}
2.1 @ResponseStatus
- HTTP Status와 Message를 제공한다.
- 개발자가 정의한 Exception이 발생하면 해당 Status와 Message를 전달한다.
- 항상 동일한 HTTP Status와 Message를 리턴하기 때문에, 같은 Exception이 발생하는 상황에서는 다른 Message를 보낼 수 없다는 단점이 존재한다.
@ResponseStatus(code = HttpStatus.NOT_FOUND, reason = "Data Not Found")
public class DataNotFoundExceptionTest extends RuntimeException {}
참고
- https://parkadd.tistory.com/70
- https://cheershennah.tistory.com/179
- https://bcp0109.tistory.com/303
- 『스프링 MVC 1편 - 백엔드 웹 개발 핵심 기술』
'Spring' 카테고리의 다른 글
RequestMappingHandlerAdapter (0) | 2021.11.16 |
---|---|
Static resources, View templates (0) | 2021.11.16 |
Controller에서 HTTP request parameter 조회하는 방법 (0) | 2021.11.15 |
Controller method parameter types (0) | 2021.11.12 |
@Controller, @RestController 차이점 (0) | 2021.11.12 |